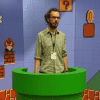
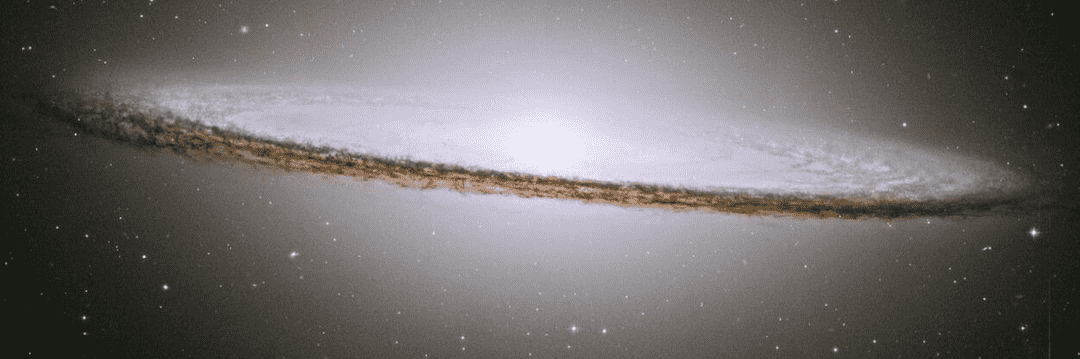
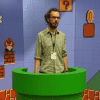
A little bit of neuroscience and a little bit of computing
This profile is from a federated server and may be incomplete. For a complete list of posts, browse on the original instance.
A little bit of neuroscience and a little bit of computing
This profile is from a federated server and may be incomplete. For a complete list of posts, browse on the original instance.
Reading Club: The Book Chs 5 & 6 "Structs and Enums" [PROJECT] ( rust-book.cs.brown.edu )
Finally, we can make our own types (or data structures)!!...
Elsevier
Alien: Romulus director Fede Álvarez clarifies new movie's timeline ( www.digitalspy.com )
Back in November 2023, star Cailee Spaeny revealed that the new movie takes place between Alien and Aliens. She wasn't wrong, but director and co-writer Fede Álvarez has clarified exactly when Alien: Romulus is set....
I miss text-based tutorials
When I use the internet to learn, I don't want to have to spend 2 minutes watching an advert, then try to decipher an accent I can barely understand whilst a 15 year old speed runs the task whilst seemingly skipping crucial steps in a video....
"Moderation tools are nonexistent on here. It also eats up storage like crazy [...] The software is downright frustrating to work with" - Can any other instance admins relate to this?
After a year online the free speech-focused instance 'Burggit' is shutting down. Among other motivations, the admins point to grievances with the Lemmy software as one of the main reasons for shutting down the instance. In a first post asking about migrating to Sharkey, one of the admins states:...
Deep Space 9 - but it's 30 Rock ( youtu.be )
What can Democrats actually do about Thomas’s and Alito’s corruption? ( www.vox.com )
[Meta] Do you have ideas for a new icon and banner?
Basically, title....
Alright, let's Fedify ( activitypub.ghost.org )
Lemmy and my Switch to PieFed ( jeena.net )
TLDR: The main reason was Lemmy hogging server resources....
Just Watched Cloud Atlas (first time) ( en.wikipedia.org )
I never got around to watching it when it came out, and I think I'd completely missed the critical reception and box-office failure it received. Which saddened me to read after the watch, I have to say, as I was really happy to have watched it....
Movie Trailers Are Killing Movies ( www.gamespot.com )
A great movie trailer can single handedly turn a movie into a success story--like that genius Cloverfield trailer in 2007 that didn't say what the title of the movie was. But it's more common these days, I'd argue, for a trailer to have the opposite impact. A generic trailer can so thoroughly dampen hype for a film that...
Rust for Lemmings "Reading Club" Alternate Slot (18:00 UTC+2) - Session 13
What?...